After the launch of Hexium in December 2018 I have been adding more levels in patch releases, but finally after 80 levels I decided the next release would be the last to add new levels. My final level, level 85, would be the end game screen, congratulating the player on finishing the game.
I wanted a “fireworks” effect on the end game screen. Although I’ve been using Corona SDK for a few years I had never touched emitters, and this seemed like a perfect fit for them. After reading the docs on creating emitters I saw that they could be completely defined by a block of JSON, which would make it easy to reuse the fireworks display code to spawn multiple types of effects.
I created a simple mask for the first emitter using an image editing program (Adobe Fireworks – how appropriate!) and then created the JSON to define the emitter. The code to spawn a new emitter is easy, so my new effect was on screen almost immediately. The next hour was spent changing the parameters and reloading the scene until I was happy with the effect.
Next I created two more emitter masks, two more emitter JSON definitions, and then created a table to hold all of the emitters. Here is the final result:
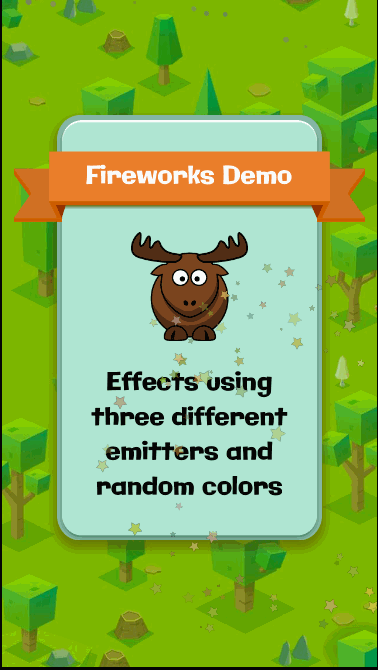
You can download all of the code from Github here: https://github.com/prairiewest/corona-fireworks All of the content in this project were either created by me or has an open license (which I have included in the licenses folder), so you’re allowed to reuse as much of it as you want.
If you’re working on a game using Corona SDK please send me a message on Twitter, I’d like to see it: @toddtrann